Cross-site scripting (XSS) is an exploitation technique that allows hackers to run arbitrary code on a compromised website. Needless to say, it is a serious risk for any web application, and our experts at Patchstack regularly receive notifications about new XSS vulnerabilities being discovered.
In this post, we will discuss what cross site scripting is and briefly explain how attackers launch XSS attacks. We will also explain how you can protect your website against XSS attacks in the future.
What is Cross-Site Scripting (XSS)?
Cross-site scripting (XSS) is a type of security vulnerability in which an attacker injects malicious code (usually JavaScript) into a web application. When this injected code is rendered on a web page, the browser automatically executes it.
This means that if a web application fails to properly sanitize or escape user input, any script tags or JavaScript code inserted into the page will be automatically executed when the page loads. The browser simply renders and runs all scripts present in the page's HTML, regardless of their origin, and the web application does not have any control over the script's execution.
The automatic nature of script execution by web browsers makes cross-site scripting a particularly dangerous security vulnerability. Malicious scripts can potentially steal user data, hijack sessions, or perform unauthorized actions without any explicit intervention from the web application.
This is a type of injection attack in which the malicious actor injects code snippets into a website’s HTML, changing how the browser renders or interacts with a page. These attacks usually target user interactions, such as submitting forms, posting comments, or clicking on links.
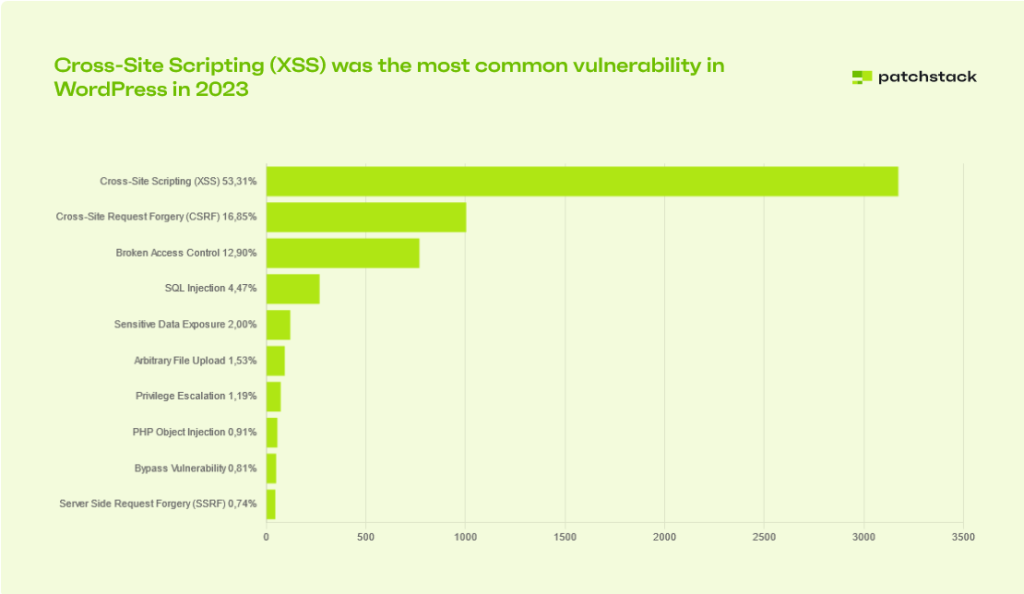
If attackers are able to successfully launch an XSS attack, then they can steal user data (e.g., login credentials, personal information) by executing scripts that capture it. This is a big deal even if the data doesn’t contain credit card information or passwords as the stolen data can be misused for identity theft, financial fraud, or other malicious purposes.
Common entry points for XSS attacks
- Input Fields: User input fields, such as search boxes, login forms, and contact forms, are common entry points. If an application doesn’t properly validate or sanitize user input, malicious scripts can be injected.
- Comments: User-generated comments on blogs, forums, or social media platforms can also be exploited. If the application doesn’t escape or filter user-generated content, attackers can inject scripts.
- URLs (Query Parameters): Query parameters in URLs can carry user input, and if an application reflects these parameters directly in the HTML response, it becomes vulnerable to XSS attacks.
- SVG Images: Unlike most images, SVG images are not fixed in size. They are built using vector graphics and scale up and down according to the screen size. Because of this, they are rendered in the user's browser, which makes SVG images vulnerable to XSS attacks.
How do XSS attacks work?
To successfully execute an XSS attack, you need to run your own code on the website’s server. Let’s take an example to understand how this is done in the wild.
Consider the following code snippet:
echo “<input type=’text’ value=’” . $_GET[‘user_input’] . ”’>”
When the above code is executed, the $_GET[‘user_input’] is populated with the response submitted by the user. Under normal circumstances, you would expect the output to look like this:
<input type=’text’ value=’*user input here*’ >
When the above code is executed, it will generate a new text field in the browser and fill it with the provided value. However, since the value of $_GET['user_input'] is controlled by the browser, it could be used to send code snippets which will be executed by the server.
For example, if the user enters 0’> <script> alert(/XSS/); </script> in the text field, then the website will generate the following code on the backend:
<input type=’text’ value=’0’> <script> alert(/XSS/); </script>’>
In the above example, the <script> alert(/XSS/); </script> portion of the page can be used to write any valid JavaScript code inside the website, which in turn gets rendered by the browser.
Once the attacker is successful, they can replace the simple alert command with malicious JavaScript code to steal information, which allows them to do anything they want.
Types of XSS
Stored XSS (Persistent XSS)
The stored XSS is the most damaging type of XSS attack as it allows the attacker to hack every single user who visits the infected webpage. Many hackers target the website's headers and footers, which are included on every page. If these components are compromised, then the hacker can attack any visitor who opens any page of the website.
When the attacker injects malicious content into a vulnerable application, its payload is permanently stored (persisted) by the application, often within a database. Now, whenever a user requests information from the website, this payload is sent along with it.
For Example: Imagine an attacker inserting a malicious script into a user input field (like a blog comment or forum post). When victims view the affected web page, all the comments are automatically fetched from the database along with the infected payload, the user’s browsers execute this infected payload which leads to unintended actions.
Reflected XSS (Non-persistent XSS)
In reflected XSS, the attacker’s payload is part of an HTTP request sent to the server but it is not stored in the database. The server reflects this payload back in the HTTP response, and then the victim’s browser executes the payload.
Attackers often use social engineering techniques (malicious links, phishing emails) to trick victims into making requests to the server. The reflected payload affects only the current victim and is not stored, which makes it comparatively less dangerous.
DOM-based XSS
DOM-based XSS is an advanced attack that occurs when a web application’s client-side scripts write data provided by the user directly to the Document Object Model (DOM). If this data is mishandled, an attacker can inject a payload.
DOM-based XSS is harder to detect because it doesn’t involve server communication.
You can learn more about DOM-based XSS in an interview I did with Rotem Bar a few months ago.
How to protect against XSS in WordPress
Keep software updated
If you are running third-party software, on your WordPress website, such as plugins or themes, you should regularly update these. Updates often include security patches that fix vulnerabilities discovered by the community or security researchers.
If you are running an older version of a theme that was previously vulnerable to XSS attacks, you can automatically protect against those attacks in the future by simply updating it when the developer releases a patch.
Web Application Firewall (WAF)
A Web Application Firewall is a protective barrier between your site and potential threats which filters incoming traffic and blocks malicious requests before they reach your application. If you properly configure the firewall on your website, then it can detect and block XSS attacks without human intervention.
The simplest way to install a firewall on your WordPress site is to install Patchstack, a WordPress security solution.
In addition to generic OWASP modules, Patchstack now provides advanced hardening for WordPress. This means you can not only block XSS attacks but also stop any attacks specifically targeted at WordPress sites, such as privilege escalation attempts and malicious file uploads.
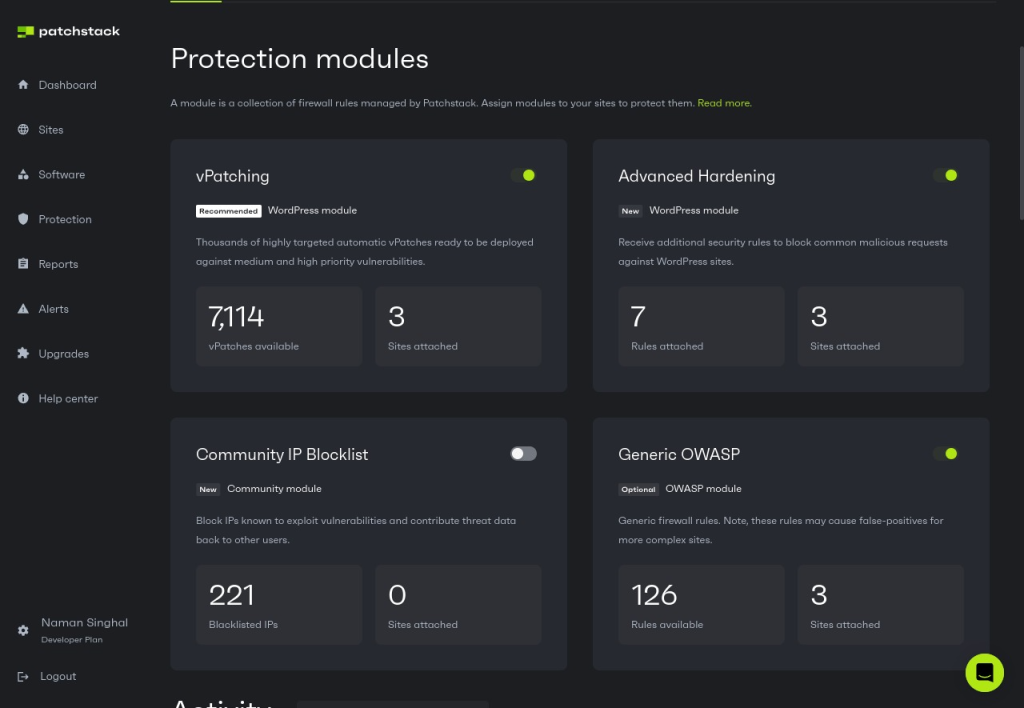
Data validation and sanitization
If you want to protect your site from XSS attacks, then there is one golden rule that you need to follow:
"Never trust user input."
If you are developing a website, then we have some good news for you – WordPress provides all of the tools you need as a developer to protect your code from XSS security bugs.
If you are writing code that accepts values from users, you should sanitize it before using it anywhere in the code.
If your code is accepting a value from the browser, before you store it anywhere, you should sanitize the value using one of the many sanitization functions WordPress has. And before you output it as HTML, be sure you escape the value again using one of the many escaping functions WordPress provides.
Which function to use depends on the value you are expecting:
- If you are storing an email address, then use sanitize_email()
- If you are expecting the name of a file, then use sanitize_file_name()
- The most commonly used function is sanitize_text_field(), which is used for … you guessed it... text fields. However, sanitize_text_field() may not provide complete protection against XSS in all contexts, particularly within HTML attributes.
For example, in an input field like <input name="aaa" value="<?php echo sanitize_text_field($value);?>">, a malicious value of bbbb" onmouseover=alert(1)" could still trigger an XSS vulnerability when a user hovers over the input.
In such cases, esc_attr() is recommended to effectively sanitize and escape values within HTML attributes. - If you want to allow certain HTML elements and attributes while protecting against XSS attacks, then you can use the wp_kses() function to filter them out
You can review all of the sanitization and escape functions in the WordPress documentation here: Data Sanitization and Escaping in WordPress.
Content Security Policy (CSP)
CSP is a security header that specifies a list of allowed resources for various content types. Whenever a web page is loaded, your browser requests a number of resources (scripts, styles, fonts, etc.) from the server. In most cases, these resources are fetched from the website server itself – but not always! Hackers can use the XSS vulnerability to fetch and execute a script from their own server on your website.
One way to fix this is by blocking all third-party resources, but if you are using a CDN to serve the images on your site or if you are loading assets from a third-party site such as Google Fonts, then these would stop working as well.
The Content Security Policy allows you to specify which websites and domains are trustworthy. Read our post on hardening WordPress to learn more.
Wrapping up
XSS attacks pose a significant threat to WordPress websites as they allow hackers to execute arbitrary code on any website. By following the recommendations provided in this article, you can protect your website against XSS attacks. If you want to learn more about this topic, we recommend checking out the guide at Excess XSS.
Protecting your WordPress website against XSS attacks is a good start, but it is not enough.
Every day, dozens, if not hundreds, of new vulnerabilities are discovered in popular WordPress themes and plugins.
If you want to stay ahead of attackers and receive a 48-hour early warning when a vulnerability is discovered, then you need to try Patchstack!